Hilarious!
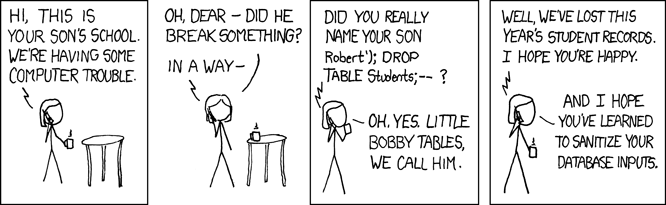
From http://xkcd.com/327/
Not to long ago I had to write a method to convert lowercase names to proper case for display on a website I was working on. I started rolling my own but then I thought that such a feature would be nice to have in the framework. So I hit google with a query and sure enough the framework authors had thought of it. The method was well hidden though ;)
CultureInfo.InvariantCulture.TextInfo.ToTitleCase("hELLo wORLd"); // Will return "Hello World"
This fits quite well in as an extension method
/// <summary> /// Creates a new string with Title Case (ie "hEllO wORLd" becomes "Hello World") using the Invariant Culture /// </summary> /// <param name="s">The string to convert</param> /// <returns>The string in title case</returns> public static string ToTitleCaseInvariant(this string s) { return ToTitleCase(s, CultureInfo.InvariantCulture); } /// <summary> /// Creates a new string with Title Case (ie "hEllO wORLd" becomes "Hello World") /// </summary> /// <param name="s">The string to convert</param> /// <returns>The string in title case</returns> public static string ToTitleCase(this string s) { return ToTitleCase(s, CultureInfo.CurrentCulture); } /// <summary> /// Creates a new string with Title Case (ie "hEllO wORLd" becomes "Hello World") /// </summary> /// <param name="s">The string to convert</param> /// <param name="ci">The culture to use when creating title case</param> /// <returns>The string in title case</returns> public static string ToTitleCase(this string s, CultureInfo ci) { if (s == null) throw new ArgumentNullException("s"); return ci.TextInfo.ToTitleCase(s); }
Now you can just call the ToTitleCase on your string objects like this:
var s = "george washington"; s.ToTitleCase();
Everyone has their set of utility programs that they need to be productive. I've got lots of them. Whenever I change computers or reinstall windows I've got a list of about 10-15 essential apps that needs to be installed before I do anything else. One of these programs is Calculator.NET by Paul Welter
It's one of those small apps that does exactly what you expect of it and nothing more. It's got a clean nice history and some built in conversion utilities but other that that it's just a calculator. It has a built in option (menu item toggle) for setting it to be the default calculator for windows. Simple, and without any hassle.
There's one tiny thing that bothers me though. At work I've got a MS Wireless Desktop 7000 keyboard with one of those neat calculator shortcut buttons right above the numpad and whenever I press it a new instance of Calculator.NET starts up. Ideally though I'd like for my existing Calculator.NET instance (if any) to be focused and restored (if minimized).
Paul was kind enough to provide the source to Calculator.NET and being a curious developer I loaded it up to see if I perhaps could add my little feature myself instead of bugging Paul with a feature request. About half an hour later I was done.
It ensures that only one instance of the calculator is active at any one time but you can override that behavior by passing -force as the first argument or by pressing CTRL+N or by just clicking on the new window icon in the toolbar/menu.
It uses a named mutex to ensure that only one instance can run at any given moment.
static void Main(string[] args) { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); string optStr = string.Empty; if(args.Length > 0) optStr = args[0].Trim().ToLower(); if (optStr != "-force" && Settings.Default["SingleInstanceMode"] != null && Settings.Default.SingleInstanceMode) { using (Mutex m = new Mutex(false, "Calulator.NET single instance")) { if (!m.WaitOne(0, false)) { // There is already an instance of Calculator.NET running. // Attempt to locate the window and bring it to front IntPtr hWnd = FindWindow(null, "Calculator.NET"); if (hWnd.ToInt32() != 0) { ShowWindow(hWnd, SW_RESTORE); SetForegroundWindow(hWnd); } } else { RunApp(); } } } else { RunApp(); } } private static void RunApp() { Application.Run(new CalculatorForm()); } const int SW_RESTORE = 9; [DllImport("user32.dll", SetLastError = true)] static extern IntPtr FindWindow(string lpClassName, string lpWindowName); [DllImport("user32.dll")] [return: MarshalAs(UnmanagedType.Bool)] static extern bool SetForegroundWindow(IntPtr hWnd); [DllImport("user32.dll")] static extern bool ShowWindow(IntPtr hWnd, int nCmdShow);
If you want to apply it to your build begin by downloading the source to Calculator.NET and then go get my patch Calculator.NET-singleinstance-patch.zip.
Just unpack the Calculator.NET zip file and then copy the contents of my zip into that directory and you're good to go. I'll drop a comment over at Paul's weblog and then we'll see if it's something he'd consider adding to the official version.
If you don't want the build hassle you can simply download the binary Calculator.NET-singleinstance.exe
Sometimes you need to get a feel for how performant a specific method is. I've seen lots of developers use DateTime.Now for this. Like this:
DateTime start = DateTime.Now; PerformWork(); TimeSpan ts = DateTime.Now - start Console.WriteLine("Time taken: {0}ms", ts.TotalMilliseconds);
Now, there's a couple of problems with this. First off, DateTime.Now performs way worse than DateTime.UtcNow since it has to get the UTC time and the calculate timezone and DST offsets.
The second and more concerning problem is that DateTime has a resolution of about 15ms, it can't be more precise than that. Now, in development code you could of course get around this by running your code a couple of thousand time to compensate for the low precision but what if you're code can't easily be run more than one time (perhaps it performs expensive and hard-to-recover database work).
The System.Diagnostics.Stopwatch class provides high-precision measurement of elapsed time. Using hardware frequency counters it achieves nanosecond precision and it's really easy to use.
Stopwatch sw = Stopwatch.StartNew(); PerformWork(); sw.Stop(); Console.WriteLine("Time taken: {0}ms", sw.Elapsed.TotalMilliseconds);
The stopwatch falls back on DateTime.UtcNow if your hardware doesn't support a high frequency counter. You can check to see if Stopwatch utilizes hardware to achieve high precision by looking at the static field Stopwatch.IsHighResolution.
This post is essentially a ripoff of my answer to a question regarding time measurement on the kickass Q/A site stackoverflow.com (not open to public yet).
I love extension methods! I really do, to the point that I have to constrain myself so that this blog won't turn into a shrine where followers of extension methods hang around all day praising the founders for giving us this precious gift.
That being said though I have one major issue with extension methods. Since exception methods is essentially glorified static methods they will accept you calling them even if the object reference is null.
string s = null; s.ToString(); // This will throw a NullReferenceException s.HtmlEncode(); // This will not
Now, did you see the difference? Since it's the string object we're talking about we all know that the HtmlEncode method doesn't belong there and thus we can deduce that it's an extension method but what if it where some lesser known object and the method name wasn't so obvious? It works since the s.HtmlEncode call will be compiled into something like this: StringExtensions.HtmlEncode(s)
Well, I thought about it for a while and I decided that I don't like it! I don't like it one bit actually and while I do respect the decision to make it this way I feel that it will essentially undermine the respect that C# developers have for null references. Whenever I look at some code calling an instance method there is something, embedded deep in my cerebral cortex, that tells me that I should watch out for nullity but when I see extension methods that allow (or actually depends on) the reference to be null my fear of NullReferenceException gradually goes away.
So what's my recommended solution? Well, with great power comes great responsibility and of course it's up to you whether or not you're going to include these methods in your code. I will not! Whenever I write an extension method I explicitly check for nullity and raise the ArgumentNullException.
The worst use of this this that I've seen so far is without a doubt the IsNull extension method which essentially lets you do this:
string s = null; if(!s.IsNull()) PerformWork(s) if(s != null) PerformWork(s)
Now do you see the problem here? It's actually more code, less obvious, (IMO) less readable than the "original" null comparison and it breaks a very important rule; you can't call instance methods on null references. Now, I have no problem with the IsEmpty extension method since that doesn't break any null rules.
PS. This whole post is actually a reaction to the anonymous comment suggesting that I convert my Collection.IsNullOrEmpty method into an extension method. DS.
Yup, you heard me. I want you to kick me. Not physically though, I hope I didn't dissapoint you there.
Under every .net related blog post I make you'll see a little image that looks like this:
That's a shortcut for kicking (or voting for) my blog post at dotnetkicks.com, a digg-like community driven site for interesting .net articles. So if you see a post that you like, please please please vote for it. I'd so like to get one of my articles onto the front page of that site. Signup is easy and I haven't had any problems with spam or unwanted email (actually, I haven't received a single email other than the confirmation mail).
Now, I'm not saying that you should vote for it if you think it stinks but if you felt that it was a well written or insightful article that someone else could appreciate I'd appreciate it if you vote for it.
C# web- and applications developer from Sweden. Hates code duplication, loves extension methods. Emerging readability evangelist